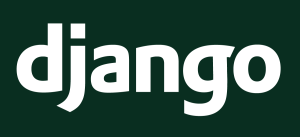
Also, these instructions assume the following:
- For simplicity's sake we're going to use SQLite for Django database - Python 2.5 and above come with SQLite support so no install necessary.
- No previous versions of Pydev modules or Django on your system.
- We'll be using the built-in versions of Python inherent in Leopard (python version - 2.5.1) or Snow Leopard(python version - 2.6)
Installing Python Support - Pydev plugin
The Pydev plugin from aptana gives you syntax highlighting, code complete, etc for Python in the Eclipse environment and in my experience work exceptionally well.
Open Eclipse and perform the following:
1) Help->Install New Software. In the "Work With" form section, input the following site to download the Pydev modules: http://pydev.org/updates.
2) Select the Pydev plugin modules in the checkbox.
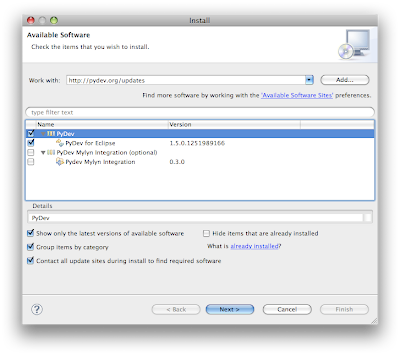
3) Click through, accepting the licensing agreements and wait for the module to install. Restart Eclipse. Open Eclipse->About and click on the Pydev Icon - we want to get the latest version of Pydev which is version 1.5.0.125.1989166 at the time of this post. If you have an earlier version use the automatic update feature of Eclipse to update just the Pydev module (Help->Update Software)
Install Django
4) Download Django 1.1.1, open a Terminal, navigate to the directory where you downloaded Django, and run the following commands:
tar -xvf Django-1.1.1.tar.gz
cd Django-1.1.1
sudo python setup.py install
Yep, it's as easy as that.
Configure PyDev to use Django
4) After installing Django, we need to ensure that the newly installed directories are visible to the Eclipse python interpreter. In the toolbar under Eclipse->Preferences->Pydev, click the Interpreter-Python option and the following window will appear:
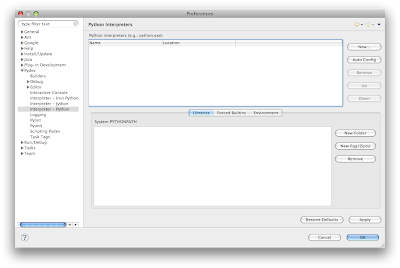
5) Click 'New' and populate Interpreter Name with any value you want (I called it 'OS X Python'), and populate the Interpreter Executable with the path to your python executable - the default path on OS X is /usr/bin/python.
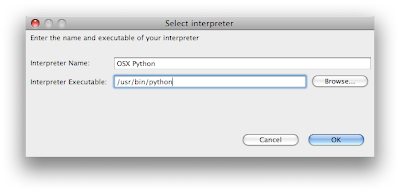
6) Eclipse will attempt to locate the libraries for Python and will display a pre-populated list of directories to add to the Eclipse PythonPath - i.e., the modules and binaries necessary for Python to run. Critical here is to select the directory where Django files were extracted when we installed it earlier. So make sure to select '/Library/Python/2.x/site-packages', as in the screenshot below. After you click 'OK' you'll be taken back to the preferences screen. Click 'OK' again and Eclipse will reparse the site packages.
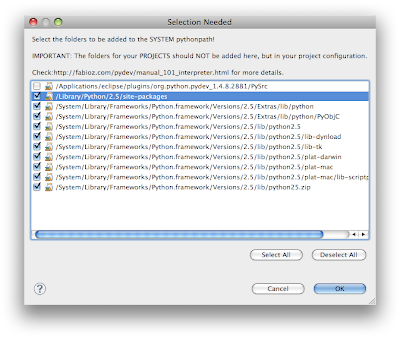
Sanity Check
7) As a quick sanity check, create a new PyDev project and then a new Python file within that project space. Test a django import statement to see if code completion is working. It should look something like the screenshot below:
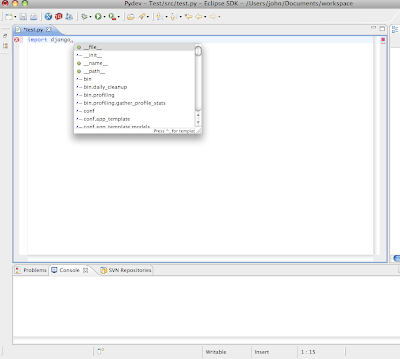
Create a New Django Project
8) Open Terminal navigate to a directory where you want to store your first Django project. Once there create a new directory for your project, navigate into the folder and enter the following command:
It's important to create the top level directory and place the new project files into a separate directory, as we'll see in a few minutes. If you navigate into the directory you created you'll see that Django has created the prepopulated files __init__.py, manage.py, settings.py, and urls.py within that directory. Now we have to import these into Eclipse.
django-admin.py startproject PROJECTNAME
9) In Eclipse, click 'File->New->Pydev Project' Name the project then uncheck 'Use Default' since we're using code that's already created for us in the previous step. Instead, click 'Browse' and navigate to the location of the top level directory you created previously. Uncheck 'Create default src folder' and click 'Finish'.
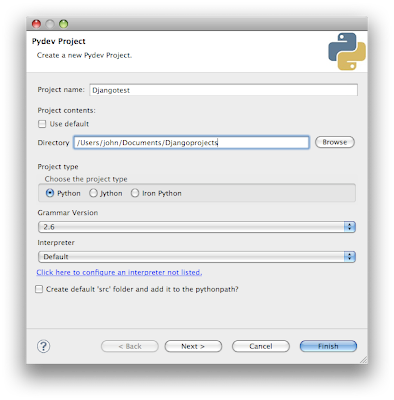
In my case, I created a top level directory named Djangoprojects and created the Django files with the django-admin.py command inside a directory named Django.
10) Within Eclipse, right-click the project you've created and click 'Properties->Pydev-PYTHONPATH->Add Source Folder' and select the project folder you created.
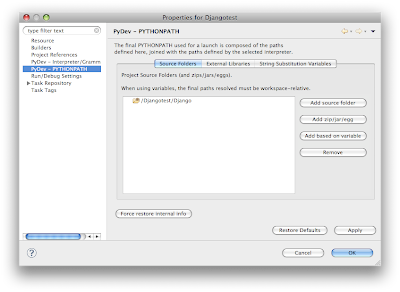
Now in the package explorer for your newly created project you'll see that your __init__.py, manage.py, settings.py, and urls.py are showing as a package.
Set up the Django Testserver
11) Now we have to configure Eclipse to start the the Django test server when we run our program. Right-click your new project in the Package explorer-> Properties->Debug Configurations(or Run/Debug Settings in newer versions of Eclipse). Click 'New' and select 'Python Run'. Press the new configuration button at the top, then give the new configuration a name (I call mine DjangoTest), under Project click 'Browse' and point to our new project, and under Main Module point to the manage.py of our new project. It should look as follows:
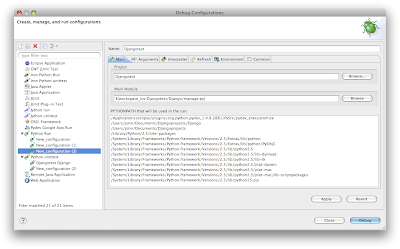
Under the Arguments tab, for Program Arguments, type in 'runserver'. Refer to the screen below:
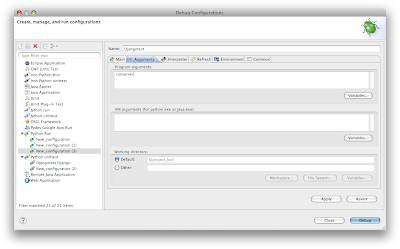
Now right-click your project, then 'Debug As->Python Run' then browse to the manage.py file within your project to launch, and click 'Ok', if all went well you'll get info in the console indicating that the pydev debugger is running:
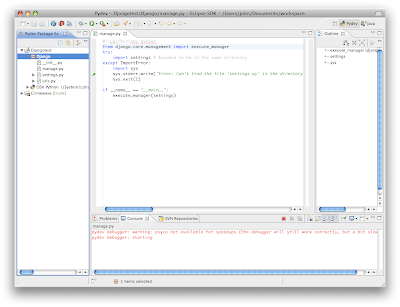
Point your browser to http://localhost:8000/ and you should see the following page:
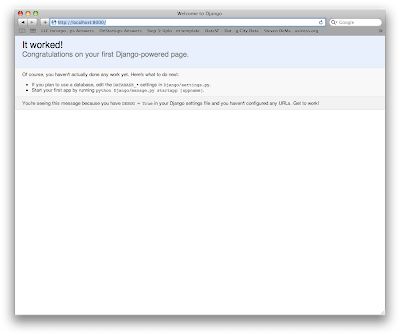
Unfortunately, you'll likely find that you're unable to shut down the server from Eclipse using the square red button near the console. Also, it won't shut down when you close Eclipse so you'll have to get in the habit of shutting down the Python process using Activity Monitor.
Debugging with Django and Python
12) Nearing the end of the process now. We have to point Eclipse to the working directory within our workspace for it to be able to access the database (SQLite in our case). So we go back to the Python perspective and right click our project->Debug As-> Debug Configurations(or Run/Debug Settings in newer versions of Eclipse)-> Arguments Tab. Under 'Working Directory' we click 'Other' then 'Workspace' and browse to our inner folder (In my case the Django folder within Djangotest). Bear in mind that if your code resides in a directory outside the Eclipse workspace you'd have to use the 'File System' button and point to the appropriate directory in your filesystem. Refer below:
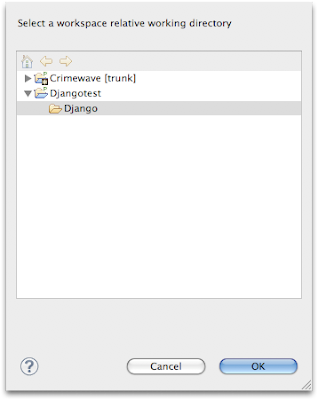
The final debug configuration should appear as the one below:
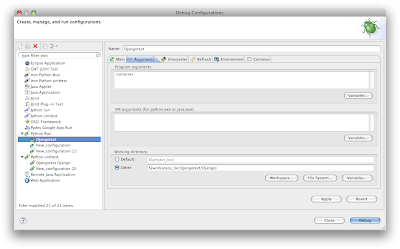
13) Lastly we need to configure Eclipse to have the python debug folder 'pysrc' in the PYTHONPATH variable. Select Eclipse in the menu toolbar -> Preferences ->Pydev->Interpreter-Python->New Folder. Browse to the plugins folder of your Eclipse install, in my case located on the path '/Applications/eclipse/plugins/org.python.pydev.debug_1.5.0.1251989166/pysrc. Click apply and Eclipse will again reconfigure the PYTHONPATH to include the debug extensions. Be careful here if you updated your pydev modules as described there will be a debug folder for the previous version and you want to make sure you're using the latest and greatest. The config should look like the screenshot below:
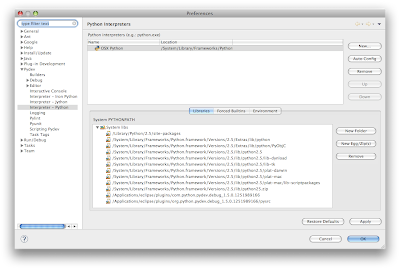
In order to simplify starting and stopping the debug server, right-click the toolbar Eclipse toolbar (within the Eclipse window) where there aren't buttons and select 'Customize Perspective'. Click the 'Command Groups Availability' and check the Pydev Debug switch. This will place the start and stop debug server options directly within the Eclipse toolbar. It should like like this:
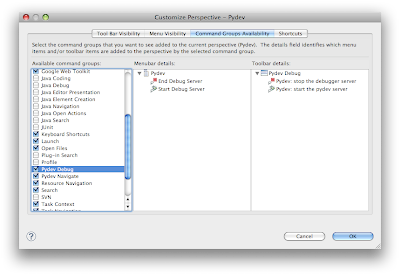
Now, when adding breakpoints to your code you'll be able to debug in Eclipse as you're used to with Java. One note: in order to see the debugging perspective within Pydev you'll have to close the Pydev perspective and reopen it so it can reload the modules we've added - otherwise you'll never see the debug option. In order to test this place a breakpoint in your python code and run the debugger. The python debug perspective will subsequently open and you'll be able to step through your code, see running values of variables, etc.
14) Celebrate. You've completed installation of Python and Django support in Eclipse and this was no easy task. I'd suggest periodically looking for updates to the Pydev extensions as they are regularly improved by the good folks at aptana. The following is a list of several resources I used to compile this post in the event that you need additional help: