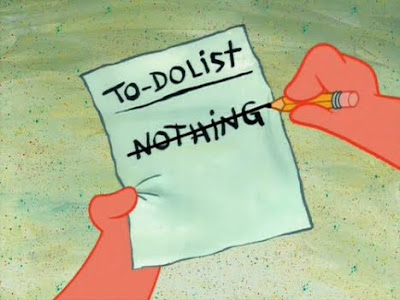
Key to coding the ListField is to register a ListFieldCallback - a class that implements the ListFieldCallback interface and can be thought of as a data structure that holds the contents of the List and permits you to modify, update, delete, and read these contents. In other words, the ListField handles the painting of the list and the Callback handles the storage of the list contents.
For this article I'll show you how to get a ListField up and running on the Blackberry and how to customize the highlighting of each row when the user scrolls across the list. For demonstration purposes we'll create the ListField as a private class within the main class that extends UiApplication and simply add our field managers that will hold the ListField to the MainScreen. Note that the code samples do not include the required code for capturing the user selection and performing an action on it - I may write another tutorial around that if people request it however that's pretty straightforward. In the end, our main goal is to have a list that looks like the one below, where the user selection is highlighted in a different color as they scroll across the list:
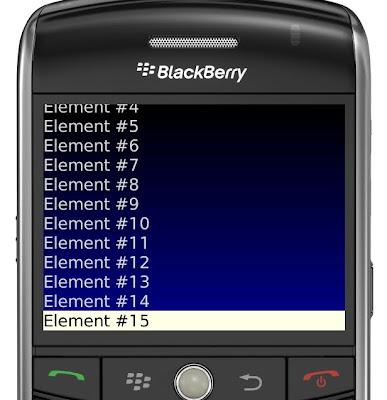
Write the Constructor
Please note that all the code below is continuous and should be contained in one file. I'll put a link to download the entire code in one file if the demand is there. However, I think it's a good learning experience to see each piece, understand how it works and put it together at the end into a cohesive working program. First, we'll import the necessary classes and create the main function for our example. I'm going to do all the heavy lifting in the constructor of the class - please note that the VerticalFieldManager and the HorizontalFieldManager in the next sections are also part of the constructor. Also, we're declaring instances of the ListField and ListFieldCallback early in the constructor and will flesh out those classes a bit later. Here's the first part of the code:
import net.rim.device.api.system.Bitmap;
import net.rim.device.api.system.Display;
import net.rim.device.api.ui.*;
import net.rim.device.api.ui.component.*;
import net.rim.device.api.ui.container.*;
import java.util.Vector;
public class ListFieldTest extends UiApplication {
public static void main(String[] args){
//main entry point
ListFieldTest theApp = new ListFieldTest();
theApp.enterEventDispatcher();
}
public ListFieldTest(){
HorizontalFieldManager _hfm;
//The _vfm will hold the ListField and we'll add it to the _hfm
VerticalFieldManager _vfm;
//Create the vars for ListField creation
ListField myList;
ListCallback myCallback;
//Get the device width and height
final int width = Display.getWidth();
final int height = Display.getHeight();
//Create the mainScreen - this holds the _hfm and _vfm managers
MainScreen mainScreen;
mainScreen = new MainScreen();
//Private class that we will create in a minute
myCallback = new ListCallback();
myCallback.erase();
myList = new MyListField();
myList.setCallback(myCallback);
//Populate the list with sample elements
for(int i=0;i<20;i++){
myList.insert(i);
myCallback.insert("Element #" + Integer.toString(i), i);
}
Create the HorizontalFieldManager
Now we need to create the HorizontalFieldManager that will hold the ListField and VerticalFieldManager. The HFM will act as the "parent" container and will hold the VFM child and we will also use it to draw the gradient. Refer to the code below for the HorizontalFieldManager:
//Draw background gradient on this manager and add VerticalFieldManager for scrolling.
_hfm = new HorizontalFieldManager() {
public void paint(Graphics g)
{
//Variables for drawing the gradient
int[] X_PTS_MAIN = { 0, width, width, 0};
int[] Y_PTS_MAIN = { 0, 0, height, height };
int[] drawColors_MAIN = { Color.BLACK, Color.BLACK, Color.DARKBLUE, Color.DARKBLUE};
try {
//Draw the gradients
g.drawShadedFilledPath(X_PTS_MAIN, Y_PTS_MAIN, null, drawColors_MAIN, null);
} catch (IllegalArgumentException iae) {
System.out.println("Bad arguments.");
}
//Call super to paint the graphics on the inherited window
super.paint(g);
}
//Sublayout is passed the width and height of the parent window and will tell the window manager
//how to layout the buttons, images, etc.
protected void sublayout(int w, int h) {
//GetFieldCount returns the number of fields attached to the instance of this manager.
//and lays out the position
if (getFieldCount() >0) {
Field searchRes = getField(0);
layoutChild(searchRes, width, height);
setPositionChild(searchRes,0,0);
}
setExtent(width,height);
}
};
Create the VerticalFieldManager
In the VerticalFieldManager declaration we will pass in the VERTICAL_SCROLL option so the lists scroll properly within the container once we add the ListField. Additionally, make sure to override the navigationMovement() function calling this.invalidate() otherwise when you scroll the ListField won't redraw as the user scrolls over the options. Try it without the invalidate() call to see what happens. Here's the final code for the VFM in the constructor:
_vfm = new VerticalFieldManager(Manager.VERTICAL_SCROLL|Manager.USE_ALL_HEIGHT|Manager.USE_ALL_WIDTH) {
public void paint(Graphics g)
{
g.setColor(Color.GRAY);
super.paint(g);
}
protected boolean navigationMovement(int dx, int dy, int status, int time){
this.invalidate();
return super.navigationMovement(dx,dy,status,time);
}
};
//Add the list to the verticalFieldManager
_vfm.add(myList);
//Add the verticalFieldManager to the HorizontalFieldManager
_hfm.add(_vfm);
//Finally, add the HorizontalFieldManager to the MainScreen and push it to the stack
mainScreen.add(_hfm);
pushScreen(mainScreen);
}//End Ctor
Create the ListField Class
Now we'll create a private ListField class and we'll override the paint() method to draw the highlight color on the selected row. The tricky part is forcing the redraw of the highlight color - you must first determine the selected row by calling getSelectedIndex() then mathematically calculate the size of the row to paint the highlight color. Here's the code (the comments are pretty detailed explaining what is going on there):
private class MyListField extends ListField{
//0,ListField.MULTI_SELECT
private boolean hasFocus = false;
public void onFocus(int direction){
hasFocus = true;
}
public void onUnfocus()
{
hasFocus = false;
super.onUnfocus();
invalidate();
}
public void paint(Graphics graphics)
{ int width = Display.getWidth();
//Get the current clipping region
XYRect redrawRect = graphics.getClippingRect();
if(redrawRect.y < 0)
{
throw new IllegalStateException("Error with clipping rect.");
}
//Determine the start location of the clipping region and end.
int rowHeight = getRowHeight();
int curSelected;
//If the ListeField has focus determine the selected row.
if (hasFocus)
{
curSelected = getSelectedIndex();
}
else
{
curSelected = -1;
}
int startLine = redrawRect.y / rowHeight;
int endLine = (redrawRect.y + redrawRect.height - 1) / rowHeight;
endLine = Math.min(endLine, getSize() - 1);
int y = startLine * rowHeight;
//Setup the data used for drawing.
int[] yInds = new int[]{y, y, y + rowHeight, y + rowHeight};
int[] xInds = new int[]{0, width, width, 0};
//Set the callback - assuming String values.
ListFieldCallback callBack = this.getCallback();
//Draw each row
for(; startLine <= endLine; ++startLine)
{
//If the line we're drawing is the currentlySelected line then draw the fill path in LIGHTYELLOW and the
//font text in Black.
if(startLine == curSelected){
graphics.setColor(Color.LIGHTYELLOW);
graphics.drawFilledPath(xInds, yInds, null, null);
graphics.setColor(Color.BLACK);
graphics.drawText((String)callBack.get(this, startLine), 0, yInds[0]);
}
else{
//Draw the odd or selected rows.
graphics.setColor(Color.LIGHTGREY);
graphics.drawText((String)callBack.get(this, startLine), 0, yInds[0]);
}
//Assign new values to the y axis moving one row down.
y += rowHeight;
yInds[0] = y;
yInds[1] = yInds[0];
yInds[2] = y + rowHeight;
yInds[3] = yInds[2];
}
//super.paint(graphics);
}
}
Create the ListfieldCallback Class
The final step is to create the private ListFieldCallback class that's attached to our ListField. Since we're not embedding images or doing other fancy things, only writing Strings to the Callback, the code is relatively straightforward, as shown below:
//Private class to populate the ListField private variable
private class ListCallback implements ListFieldCallback{
private Vector listElements = new Vector();
public void drawListRow(ListField list, Graphics g,
int index, int y, int w) {
String text = (String)listElements.elementAt(index);
g.setColor(Color.LIGHTGREY);
g.drawText(text, 0, y, 0, w);
}
public Object get(ListField list, int index) {
return listElements.elementAt(index);
}
public int indexOfList(ListField list, String p, int s) {
//return listElements.getSelectedIndex();
return listElements.indexOf(p, s);
}
public void insert(String toInsert, int index) {
listElements.insertElementAt(toInsert, index);
}
public void add(String toInsert){
listElements.addElement(toInsert);
}
public void erase() {
listElements.removeAllElements();
}
public int getPreferredWidth(ListField listField) {
// TODO Auto-generated method stub
return 0;
}
}
}
The final result will be a scrollable list that highlights the user selected option in a Light Yellow color, as shown in the screenshot at the top of this post. Once you understand how this works you can see how modifications to the overridden paint() method in ListField could permit you to have highly modifiable rows in the list - for example, in a Flikr client app you might have a scrollable list of your friends with a thumbnail on each row showing a sample picture from that friend's account. When the user scrolls to a new friend in the list (startLine == curSelected), you might initiate a function that updates the thumbnail with a changing feed of images from that user's account. The possibilities are almost infinite when customizing ListFields. I'll be on the lookout for questions in the comments section.
15 comments:
This is a fantastic post, and has helped me a lot to better understand custom list fields. I appreciate your work in writing this up. One question I have, if you don't mind commenting on, is how to access the list field and update/remove elements. For example I have a thread that runs every 10 seconds, and I would like that thread to update the list field with new data. Any ideas would be appreciated. Thanks for the help.
Hey Sean,
Thanks a lot for your comments. I thought your question was really good and merited an article in and of itself so check it out: http://berrytutorials.blogspot.com/2009/12/blackberry-threads-dynamically-update.html. The sample code shows how to add elements from a thread but not how to remove them - I think you'll be able to use what's there to also remove elements pretty easily.
This is a great site with great examples. Thanks. My question is how can you change the row height of the currently selected row and also set the last row back to the default height? Any help would be greatly appreciated.
@Anonymous: Thanks for the comment. To change row height you have to look at the ListField class that my 'custom' listfield is extending. I may be misunderstanding your question but to adjust the height of a row in the custom listfield you'd use the base ListField classes methods - e.g., setRowHeight()
This is a great example. I think I may have a simpler solution. It seems to work, but I don't have a lot of experience in Blackberry UI, so I may have missed some major flaw. I just override ListField.drawFocus like this:
protected void drawFocus(Graphics graphics, boolean on) {
XYRect rect = new XYRect();
getFocusRect(rect);
if (on) {
graphics.setColor(Color.DARKRED);
graphics.fillRect(rect.x, rect.y, rect.width, rect.height);
graphics.setColor(Color.WHITE);
getCallback().drawListRow(this, graphics, getSelectedIndex(), rect.y, rect.width);
}
}
The call to getFocusRect returns the rectangle surrounding the selected row. I fill it with dark red and then draw the row content. Some caveats. I needed to explicitly set the row height. Otherwise the system seems to add a small vertical offset to y when it calls drawListRow. I think this is an attempt to vertically center text in the row. I've only tried this with single select list fields. Not sure what would happen for multi-select.
Thank you for the great post. This has been a very useful example for furthering my understanding of listfieldcallback. One problem I cannot resolve is running this on a Blackberry 9500 (Storm) simulator. I start the application and rotate the device from portrait to landscape and the fields do not repaint. Other field types and managers repaint properly as long as I use the USE_ALL_WIDTH style, but I have not found a solution for the custom listfield.
Does anyone know how to expand and collapse list fields??
Great example of the ListField api John. I tried this out using the 5.0 JDE with the default Touchscreen simulator and it works as you describe. However I noticed when I did a right click up or down mouse movement that simulates a finger up or down swipe the gradient in the horizontal field manager does not repaint. Is there a solution to that problem?
Rich,
thanks a lot.. the getFocusRect helped a great deal :))
How would you go about selecting one of these list items that leads into another list?
Thanks,
Mike
I got proper understanding customization list..
Thanks
Satish
"Additionally, make sure to override the navigationMovement() function calling this.invalidate() otherwise when you scroll the ListField won't redraw as the user scrolls over the options. Try it without the invalidate() call to see what happens." - Great hint for implementing any custom field/manager. I suffered a lot for that, Thank you.
i am new to blackberry. i want to make a an application including list, like
value1 : ____
value2 : ____
Calculate
<---now my list--->
+ : (result)
- : (result)
* : (result)
/ : (result)
<---End of list--->
Is it possible to make a list like this?
thank u rely helpful
Thanks Man.
You Save My Life :)
Post a Comment